mobile
Dummies Guide to Delegation in Swift
Want to know how delegation in swift is implemented? Here is the step-by-step process you can follow to implement and know-how delegation works in swift

Karthik Kamalakannan / 12 August, 2019
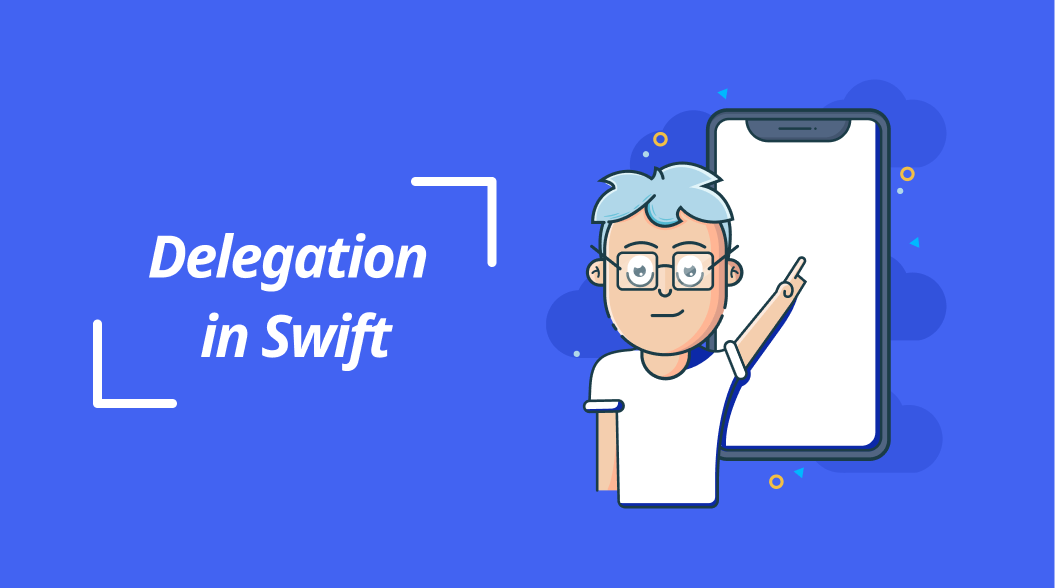
The delegate patterns have been there for a very long period on the Apple platform. Delegation is mainly used for handling table view events using UITableViewDelegate, to modify cache. The core purpose of a delegate is to report back to the owner in a decoupled way.
What exactly is Delegate?
Delegates are a design pattern that allows one object to send messages to another object when a specific event happens.
Consider an Object A calls an Object B for action and A should know that B has completed the action. This can be easily achieved with delegates
How to create a Delegate?
I'm going to show you how to create a custom delegate that passes between classes.
Consider having two classes ViewControllerA and ViewControllerB. A has a UILabel and a Button and B has TextField & a Button. Clicking on a button in A will navigate to B. When the user enters a data in the TextField,it will return back to A when the user clicks the Button in B.
Step 1 : Create a Protocol in ViewControllerB
Protocol ViewControllerBDelegate : Class {
func textFromTextField( textData: String )
}
This is the first step towards creating a delegate. You can implement as many functions you want depending on the requirement. In our case, we need only one function that accepts the string.
Step 2 : Create a delegate property in ViewControllerB
weak var delegate : ViewControllerBDelegate ?
We must create delegate property for the class and give protocol ViewControllerBDelegate as the type and it should be optional. And you should add the weak keyword to avoid memory leaks.
Step 3: Add Delegate method call in tap event in ViewControllerB
delegate?.textFromTextField(text);
This should be added in tap event of the button which takes back to ViewControllerA.
That contains a text which is from the TextField in ViewControllerB.
Step 4 : Import delegate in ViewControllerA
Class ViewControllerA : UIViewController, ViewControllerBDelegate {
Now ViewControllerA has taken ViewControllerBDelegate protocol but the compiler will throw an error saying "Type ViewControllerA does not conform to protocol ViewControllerBDelegate__" this means you didn't use the protocol in ViewControllerA.
We must use all the functions from the delegate. Adding the function to class ViewControllerA that are added in ViewControllerBDelegate will remove this error.
Step 5 : Assign ViewControllerB to ViewControllerA
If you are using segue for navigation, bind it through the prepareforsegue method.
if let nav:segue.destination as? UINavigationController, let ViewControllerB:nav.topViewController as? ViewControllerB {
ViewControllerB.delegate:self
}
if you are using delegate within a ViewController (Like data transition between ViewController and UIView). Add the below code in viewDidLoad of ViewControllerB
var vc :ViewControllerA();
Self.delegate:vc as! ViewControllerBDelegate
Step 6: Finally use the method of the ViewControllerBDelegate
func textFromTextField(textData : String) {
displayLabel.text:textData;
}
Every time you click on the Button in ViewControllerB, you will get the textData String from the textFromTextField function.
Conclusion
Delegation is an important design pattern to understand if you want to become an iOS developer. Delegation touches on many principles of good app architecture and proves its uselessness even though it is an old design pattern.
Last updated: January 23rd, 2024 at 1:50:36 PM GMT+0