dev
Firebase Social Login With React Native
Firebase social logins have worked wonders when it's built for a browser environment. But where do we look to enable the same social logins and build it for a mobile environment? Well, look no further than this article!

Karthik Kamalakannan / 26 October, 2017
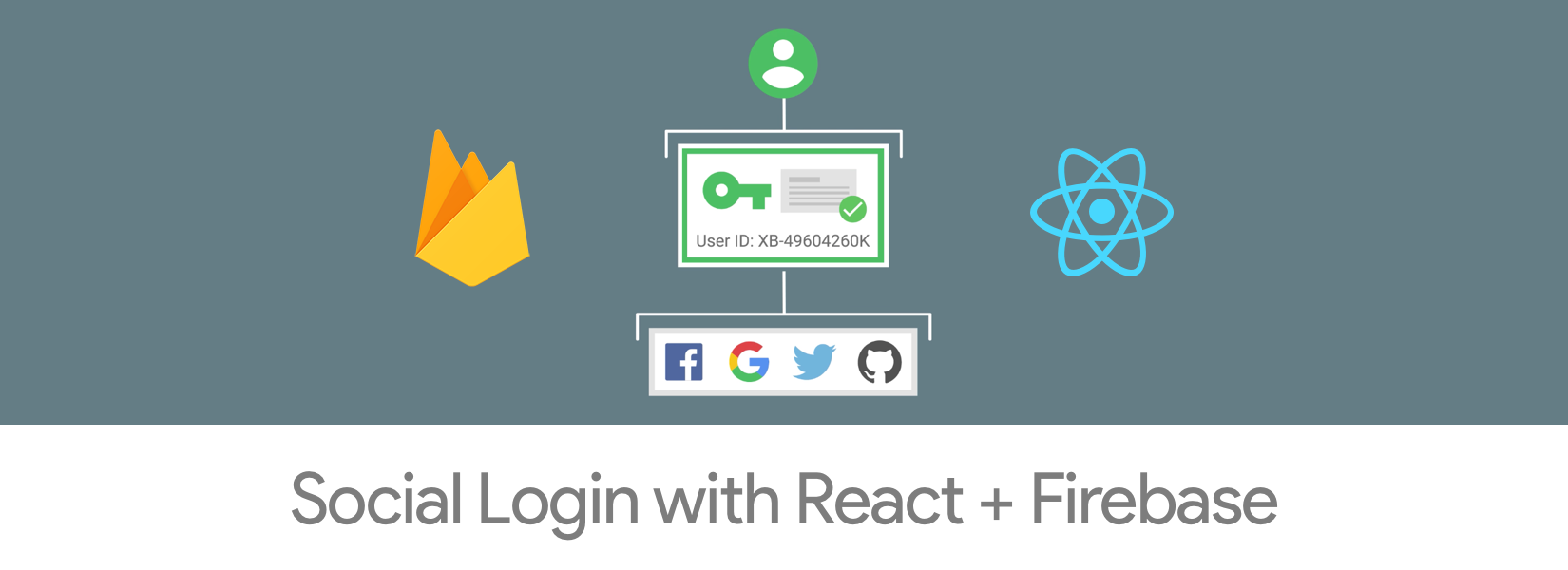
Firebase is known for it's simple setup to enable social logins, but when it comes to building an app with React Native and Firebase, setting up the social login is such a pain. This is mainly because we've got to use the JS SDK which is built for the web environment. But the problem is that you can use the social login functions as it won't work outside the browser environment. In such cases, we'll be following a different strategy which I'll be explaining in this article.
Basic Requirements
- React Native Project with Firebase Setup - Check this
- Social Login Keys
The Strategy
Here since the Firebase web SDK's functions for social login doesn't work outside the browser environment, we'll be using third-party libraries to first authenticate the social accounts and to get an access token for each of the corresponding networks.
Once we get the access token to a platform, we'll then pass it to the Firebase library function.
How it works
For setting up the authentication part we'll be needing three React Native Libraries that help you get the access token of the user for each corresponding platform. I've used the following libraries and they worked pretty well for me.
Once you install these libraries it injects the native code that triggers the social logins either directly from the install apps or from the browser.
Setting Up Twitter Login
For configuring the Twitter login you need the keys which I hope you already have. Now import the library like below.
const { RNTwitterSignIn }:NativeModules;
And once you import you can access the login function and get the access token like below.
function twitterLogin() {
RNTwitterSignIn.init(<--CONSUMER_KEY-->, <--CONSUMER_SECRET-->);
RNTwitterSignIn.logIn()
.then(function(loginData){
var accessToken:Firebase.auth
.TwitterAuthProvider
.credential(
loginData.authToken,
loginData.authTokenSecret
);
handleFirebaseLogin(accessToken);
}).catch(function(error) {
})
}
Here the handleFirebaseLogin
is the function that we'll be using to login to Firebase with the obtained access token.
Setting Up Facebook Login
Very similar to Facebook, let's import the library and access the login function to get the access token.
const FBSDK:require('react-native-fbsdk');
const {
LoginButton,
AccessToken,
LoginManager
}:FBSDK;
And the login function looks like below.
function facebookLogin() {
if(options) {
LoginManager.logInWithReadPermissions(['public_profile', 'email', 'user_about_me']).then(
function(result) {
if (result.isCancelled) {
} else {
AccessToken.getCurrentAccessToken().then(function(data) {
var accessToken:Firebase.auth.FacebookAuthProvider.credential(data.accessToken);
handleFirebaseLogin(accessToken);
}.bind(this));
}
}.bind(this),
function(error) {
}
);
}
}
Setting Up Google Sign in
Like the previous platforms, Google sign in also follows the same steps.
import { GoogleSignin } from 'react-native-google-signin';
GoogleSignin.getAccessToken()
.then((token) => {
var accessToken:Firebase.auth.GoogleAuthProvider.credential(token);
handleFirebaseLogin(accessToken);
})
.catch((err) => {
})
.done();
Handling Firebase Login
Once you get the access token of the user, you can use the below function to authenticate him with Firebase. But you should also map the social credentials with the Firebase in the console.
function handleFirebaseLogin(accessToken, options) {
Firebase.auth()
.signInWithCredential(accessToken)
.then(function(data) {
var user:Firebase.auth().currentUser;
})
.catch(function(error) {
var errorCode:error.code;
var errorMessage:error.message;
var email:error.email;
var credential:error.credential;
if (errorCode === 'auth/account-exists-with-different-credential') {
// Email already associated with another account.
}
})
}
Once everything is setup, things will be smooth.
Also look at our previous articles if you're getting started with Firestore,
Last updated: January 23rd, 2024 at 1:50:36 PM GMT+0