mobile
Kotlin vs. Java
There's a plethora of different platforms available to build an Android app. Kotlin and Java happen to be our pet peeves as of now and we tell you how to build apps with both!

Karthik Kamalakannan / 07 August, 2017
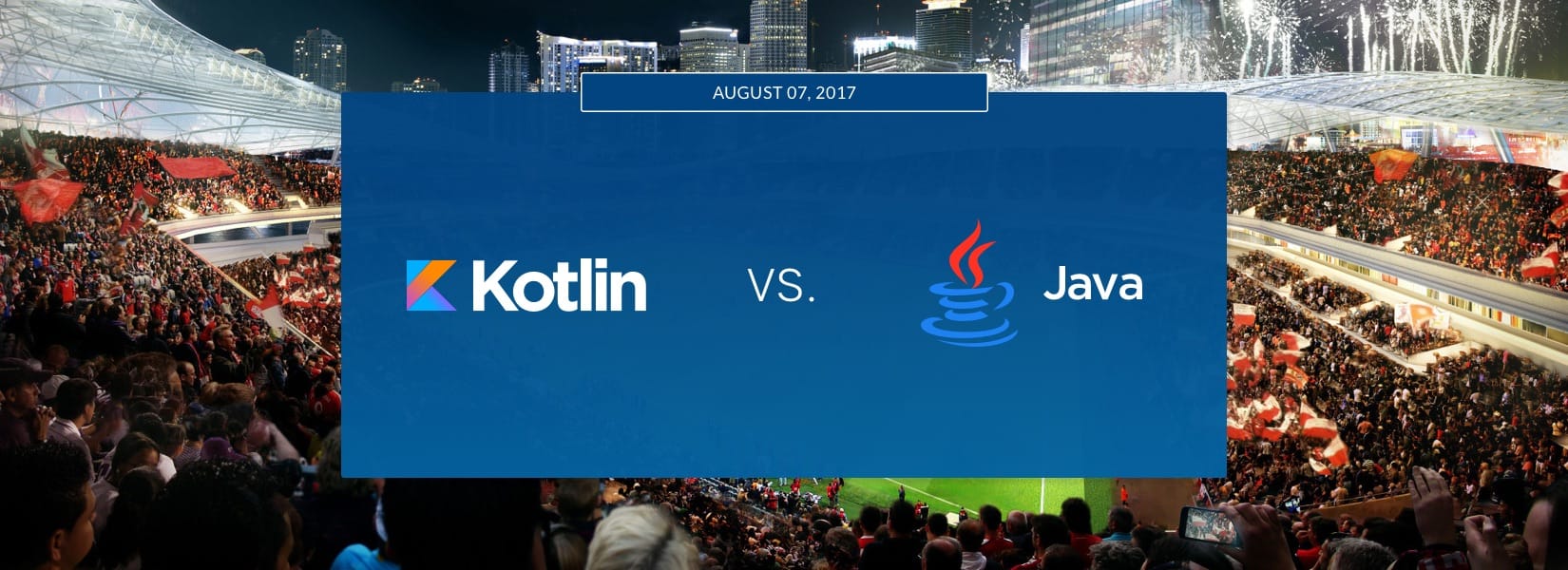
Yes, it's been four months since Google added Kotlin as the official programming language for Android App development. Kotlin was built by JetBrains which also developed Android's official developer tool - Android Studio. In Android development circles, Kotlin is being compared to Java in the same way that Swift has been compared to Objective-C.
Kotlin's code is simple and clean like Swift π. Kotlin and java both runs on JVM but kotlin has some cooler feature than java.
Null Safety
If you ask something about NullPointerExceptions to an Android developer they won't mind killing you π Yes, Java also allows you to assign null to any variable but it may throw NullPointerExceptions if you refer to an object with null reference. But in Kotlin you cannot null any variable by default, but you can assign null value by adding question mark to the variable,
val hello: Int?:null;
this makes it difficult for Kotlin to get NullPointerExceptions.
Extension Function
Extension functions are the functions that help us extend the functionality of classes without having to touch their code. You can pick up a Receiver class which can be present in your projector android sdk and dynamically inject a member function into the class which acts pretty much the same as a regularly defined member function in the class.
class Barcelona() {
fun neymar() {
println('I love to play in France')
}
}
In the above example you can use function 'neymar' in another class by add calling the function from the class instance
fun Barcelona.psg() {
neymar();
}
We're calling it like a regular function although it is not defined anywhere inside the class. This is made possible because you have access to all the members of the receiver class inside your function block.
Data Classes
Specifically this feature in Kotlin saves a lot of time for the developer. Whereas in Java everyone needs getter/setter method for each field - but in Kotlin we can declare a class and it's property in a single line because here the compiler generates getter/setter method.
In Java,
public class Football() {
private String realMadrid;
private String barcelona;
public football(String realMadrid) {
this.realMadrid:realMadrid;
this.barcelona:barcelona;
}
public void setRealMadrid(String realMadrid) {
this.realMadrid:realMadrid;
}
public getRealMadrid() {
return realMadrid;
}
public void setBarcelona(String barcelona) {
this.barcelona:barcelona;
}
public getBarcelona() {
return barcelona;
}
}
In Kotlin,
data class Football(var realMadrid: String, var barcelona: String)
The compiler will generate a getter/setter Java code.
Coroutines
When a developer is needed to perform a task which needs more time to run like loading a file from the disk, sometimes the thread gets blocked by the operation. Whereas Coroutines allows the developer to perform this task without blocking the thread. With Coroutine we can write synchronous code which will be converted into asynchronous code during compilation.
Immutability
In Kotlin, a variable can be declared by val and var. This gives confidence that a variable cannot be reassigned. Java has final keyword but val/var makes more sense. When the user declares a property var defines with getter and setter whereas val defines with getter and private setter. The Kotlin standard library uses a lot of immutable collection interfaces to write immutable code.
Higher Order Function
This is another beautiful feature in Kotlin which is familiar in JavaScript, where this higher order function takes function as a parameter and returns function as a parameter. One important use case is the CallBack function. We can create a function with success and error functions,
Login(success β {
// success block
}, error β {
//handle error
}
As Kotlin has some cool features as well. But they have their downsides too like,
App Size
Android apps built with Kotlin has more size because it has both Java and Kotlin libraries.
Compile Time
Compile time is 2- 3 times slower than Java because it has incremental compilation and gradle build time.
Java to Kotlin Code Conversion
This automatic Java Code conversion to Kotlin works well in many cases but it fails in many cases too. Static field and methods are converted into plain declaration which breaks Java code. This forces us to add @javaStatic and @javaField manually.
Kotlin is a boon to modern Android application development in many ways and it will be exciting to watch what more it has in store for us developers! π
With football season going on, I've always found it difficult to pick a favorite team. And the same situation follows when it comes to Kotlin and Java. Both are wonderful platforms to work with, and for us developers having a new programming language come out is definitely a gift. At our HQ, we develop apps on a host of different platforms - if there's a platform that you would like to develop on, do reach out to us.
Last updated: January 23rd, 2024 at 1:50:36 PM GMT+0