dev
Here's how to send Slack notifications using Firebase Cloud Functions and FireStore
With the release of FireStore we can now use it with cloud functions to do lots of wonders. Now, we are going to create a simple slack notification system via webhooks. Learn More.

Varun Raj / 06 October, 2017
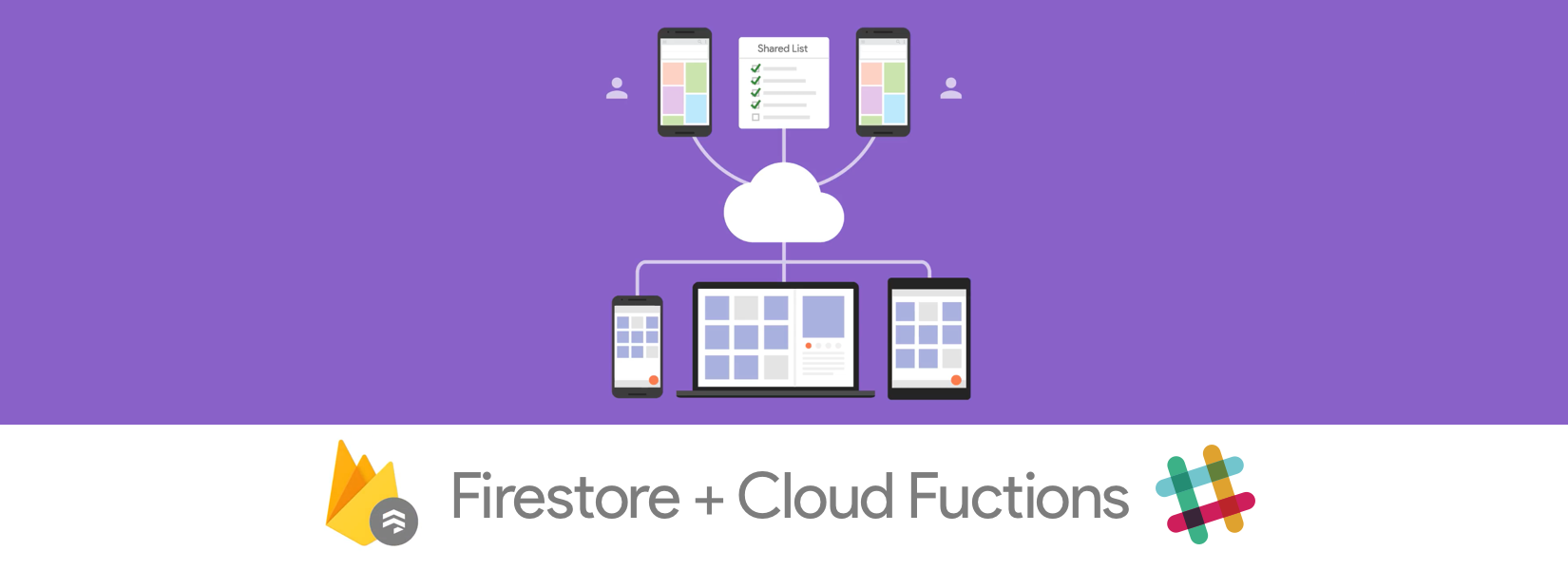
In our previous article we saw about how to kick start with Firebase's new add-on FireStore. Now let's see how we can use Firestore along with cloud functions to perform a lot of background operations as well. In our use case, we'll be creating a simple Slack notification system where the todos are created and their statuses are updated.
If you landed here directly I'd strongly recommend you to look at our previous article Getting Started with Cloud Firestore in 5 Minutes to get more information and clarity since we'll be using the same example.
Right now we've a todo list where we can create and toggle status of a todo. With this example, we'll be sending notifications to a Slack channel where a new todo is created or when a todo is marked as checked/unchecked.
Few Basic Setup.
First you need to get a Slack webhook in order to send messages to a channel or a group in your workspace. For creating the webhook follow this link.
Once the webhook is created, note it down and we'll be using that to configure Firebase's cloud function.
Firebase Cli
If you haven't updated the firebase-tools
before, update it with the following command.
npm install -g firebase-tools
Now create a Firebase project in folder with the following command.
firebase init
Now select Firebase functions in the menu popped in terminal, once complete you'll find a folder called functions
and other configuration files.
Writing Cloud Functions
Now open the index.js
file inside functions folder. this is the entry point for our cloud functions and we'll be writing our functions here for this example.
Installing Slack SDK
Also install the Slack node sdk in the functions folder.
npm install @slack/client --save
Configure Slack
We'll be using the webhook url that we got from Slack for configuring the sdk.
var IncomingWebhook:require('@slack/client').IncomingWebhook;
var url: "<YOUR--SLACK--WEBHOOK--URL>";
var webhook:new IncomingWebhook(url);
Function to send message to Slack
We wrote a common function that we'll be using to post messages to Slack passing the message string as parameter
function sendMessage(message) {
webhook.send(message, function (err, header, statusCode, body) {
if (err) {
console.log('Error:', err)
} else {
console.log('Received', statusCode, 'from Slack')
}
})
}
Writing Cloud functions
First, in order to listen to a specific document we'll be using the following pattern
exports.functionName:functions.firestore.document('todos/{todoId}')
The cloud functions for Firestore is very similar to that for realtime databases. We'll be using two triggers - onCreate()
and onUpdate()
for triggering when new todo is created and when a todo's status is toggled.
On Create
exports.createTodo:functions.firestore.document('todos/{todoId}').onCreate(event => {
var newValue:event.data.data();
var message: "New Todo Added : " + newValue.title;
sendMessage(message);
return true;
});
On Update
exports.updateTodo:functions.firestore.document('todos/{todoId}').onUpdate(event => {
var newValue:event.data.data();
var message;
if (newValue.checked) {
message:newValue.title + " is marked as checked";
} else {
message:newValue.title + " is marked as unchecked";
}
sendMessage(message);
console.log("Udpated Todo : ", JSON.stringify(newValue));
return true;
});
Also do checkout our other articles on Firebase here:
Last updated: January 23rd, 2024 at 1:50:36 PM GMT+0