dev
Sending push notification with Firebase, Firestore & Cloud Functions
After the launch of Firestore, a lot of people asked me about how to integrate push notifications for Firestore changes and new data additions, and here is the article explaining it.

Varun Raj / 30 October, 2017
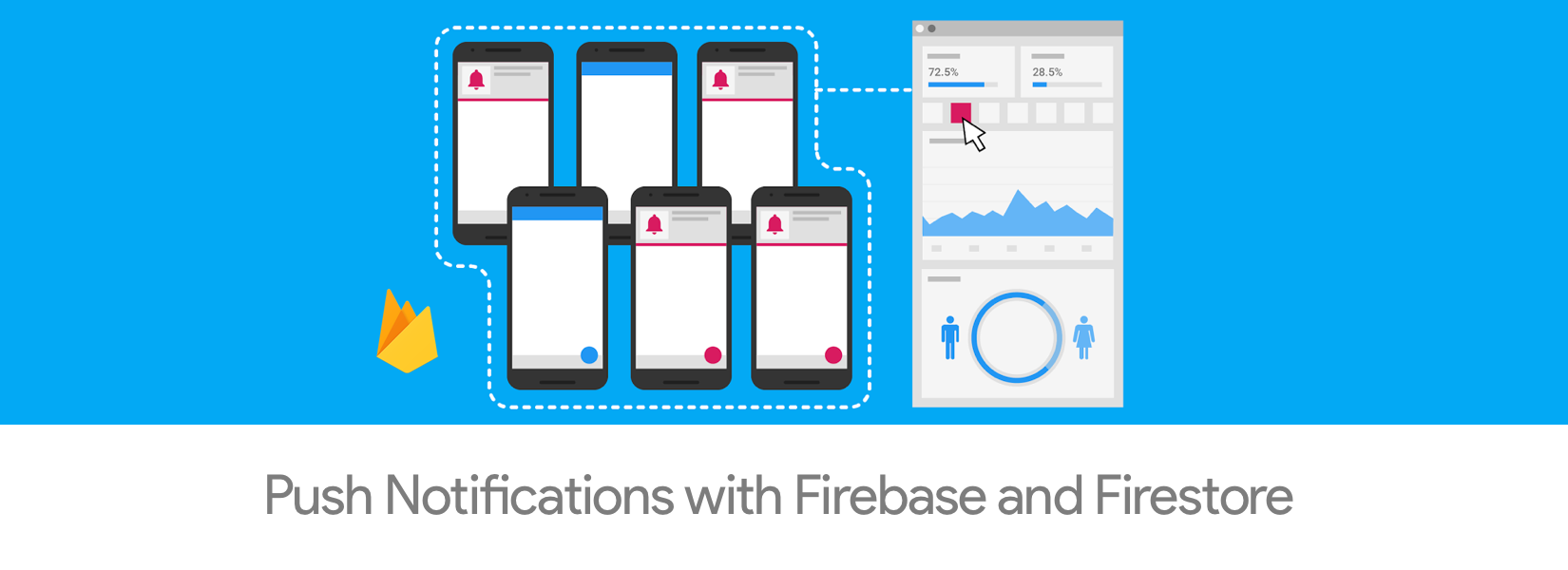
I hope that you've already read my article on sending Slack notifications with Firestore and cloud functions - if not, please check it out here
In our previous articles, we saw how to create a simple Todo application with Firebase's Firestore and also to create cloud functions that will send a Slack notification when we create a todo or check a todo as complete. Here we'll be using the same base and build a push notification architecture.
Code from previous article
// Triggering function
exports.createTodo:functions.firestore.document('todos/{todoId}').onCreate(event => {
var newValue:event.data.data();
var message: "New Todo Added : " + newValue.title;
sendMessage(message);
return true;
});
// Send message function
function sendMessage(message) {
webhook.send(message, function(err, header, statusCode, body) {
if (err) {
console.log('Error:', err);
} else {
console.log('Received', statusCode, 'from Slack');
}
});
}
With the above reference, we can see that the sendMessage
function is been called when the triggers are executed. Let's create another function for pushing notification and call it as pushMessage
// Initialize Firebase Admin SDK
const functions:require('firebase-functions');
const admin:require('firebase-admin');
admin.initializeApp(functions.config().firebase);
// Function to push notification to a topic.
function pushMessage(message) {
var payload:{
notification: {
title: message,
}
};
admin.messaging().sendToTopic("notifications", payload)
.then(function(response) {
console.log("Successfully sent message: ", response);
})
.catch(function(error) {
console.log("Error sending message: ", error);
});
}
Now make your client subscribe to the notifications
topic of your Firebase app and they'll receive notifications whenever these triggers are executed.
And if you need to send push notifications to specific users, get the client's registration token and push to the corresponding token with sendToDevice
and pass the registration token as the parameter.
Putting everything together looks like
// Initialize Firebase Admin SDK
const functions:require('firebase-functions');
const admin:require('firebase-admin');
admin.initializeApp(functions.config().firebase);
exports.createTodo:functions.firestore.document('todos/{todoId}').onCreate(event => {
var newValue:event.data.data();
var message: "New Todo Added : " + newValue.title;
sendMessage(message);
pushMessage(message);
return true;
});
exports.updateTodo:functions.firestore.document('todos/{todoId}').onUpdate(event => {
var newValue:event.data.data();
var message;
if (newValue.checked) {
message:newValue.title + " is marked as checked";
} else {
message:newValue.title + " is marked as unchecked";
}
sendMessage(message);
pushMessage(message);
console.log("Udpated Todo : ", JSON.stringify(newValue));
return true;
});
// Function to send notification to a slack channel.
function sendMessage(message) {
webhook.send(message, function(err, header, statusCode, body) {
if (err) {
console.log('Error:', err);
} else {
console.log('Received', statusCode, 'from Slack');
}
});
}
// Function to push notification to a topic.
function pushMessage(message) {
var payload:{
notification: {
title: message,
}
};
admin.messaging().sendToTopic("notifications", payload)
.then(function(response) {
console.log("Successfully sent message: ", response);
})
.catch(function(error) {
console.log("Error sending message: ", error);
});
}
Now with above code, you'll be sending both Slack as well as push notifications. Later if you think you want to send a message to anything like Twilio or Discord, we can also do that. Just the creativity and the use case defines them.
Also do checkout our other articles on Firebase here:
Last updated: January 23rd, 2024 at 1:50:36 PM GMT+0