frontend
Setting up Browserify and React, on Rails
Our Sr. Architect explains how you can integrate Browserify and Rails with React.

Varun Raj / 30 July, 2016
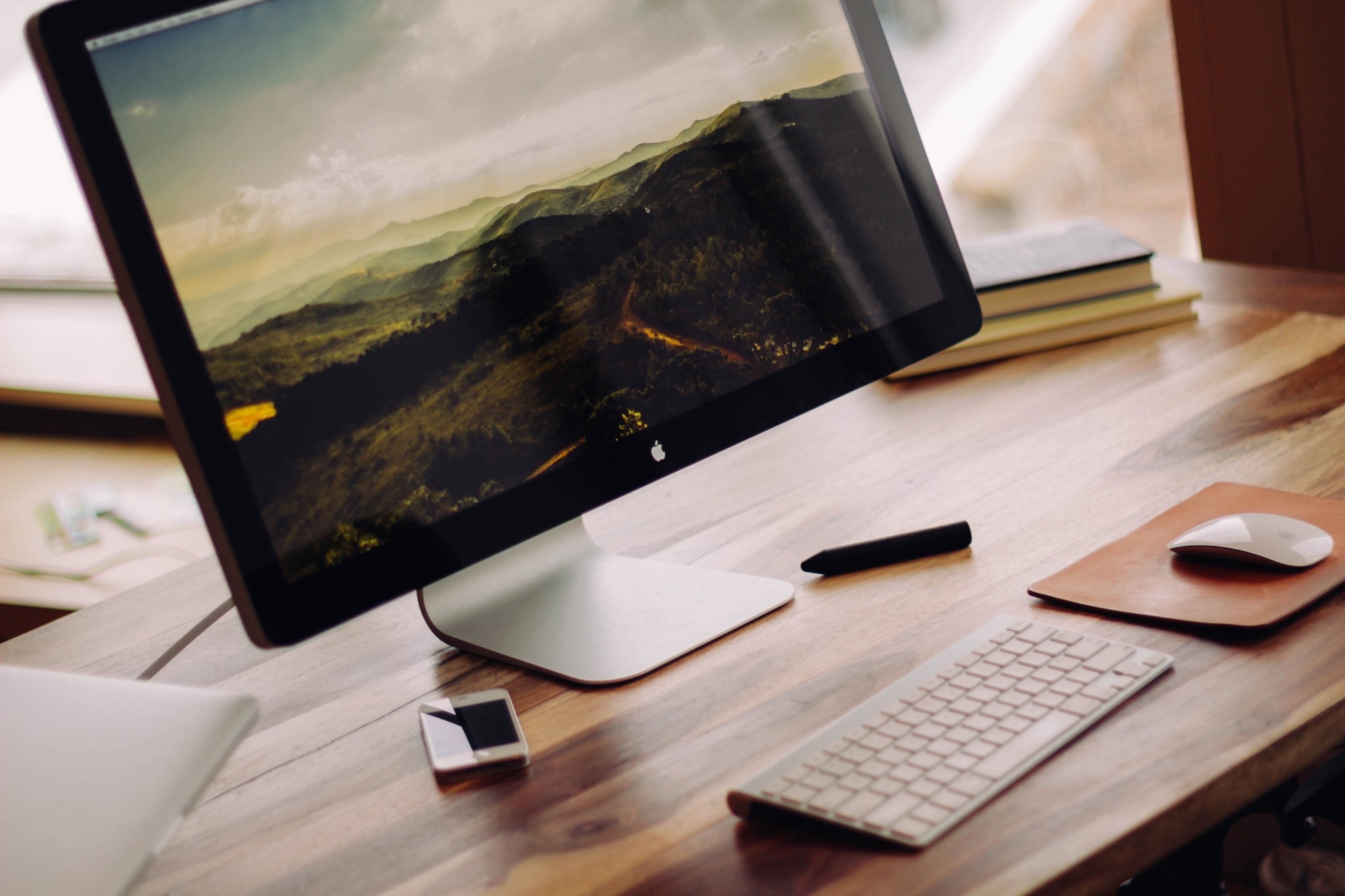
Rails is such a brilliant framework, it's got almost all the possible features inbuilt. However, even though it has a lot of support for front end libraries and frameworks, it lacks support for node packages. We need to rely on a third party tool called Browserify, for this.
So I thought of trying it, but it wasn't as easy as I thought. I failed many times. Sometimes I thought of dropping the idea, but my curiosity never let me down. One fine day I finally integrated it. And this is how I did it,
Create a Rails project (or use one you already have)
As a start, create a rails project of your desired name.
rails new app_name
Add the following gems to the Gemfile.
# React and NPM
gem 'react-rails'
gem 'browserify-rails'
Note: react-rails will be used for integrating and using React components directly in ERB files.
Note: browserify-rails will be used for integrating Browserify into the Rails assets pipeline.
And then run this,
bundle install
Now everything is set to configure Browserify.
In your config/application.rb add the following.
config.browserify_rails.commandline_options: " — transform reactify — extension=\".jsx\""
This is to instruct the Rails app on how to bundle your npm modules and add them to the asset pipeline.
Setting up npm modules
The first step to set up npm in your rails app is of course to initializing it.
npm init
This will ask you a set of configuration questions, once completed it will create a package.json file in your root folder. This package.json is like a Gemfile, but for Node. Read more about it on the npm website.
Now its time to add our core dependencies and devDependencies for node. As we're going to develop on React, we'll add it also as a dependency.
"dependencies": {
"react": "^15.2.1",
"react-dom": "^15.2.1",
"browserify": "^12.0.2",
"browserify-incremental": "^3.1.1"
},
"devDependencies": {
"babel-preset-es2015": "^6.6.0",
"babelify": "^7.2.0",
"reactify": "^1.1.1"
}
Here, the packages I added under devDependencies are used to transform of Javascript into a way that can run on the browser.
Now run the following command to install all the packages to your Rails app.
npm install
You can see that a new folder has been created in the root folder of your Rails app named node_modules, this is where all your npm packages go after installation.
After installing all the required modules, create the following files and folder under your app/assets/javascript.
A File
components.js
A Folder
components/
components.js is the file where you'll be referring all your major and global components with Browserify's *require *method. And components is the directory where we'll place all our custom crafted components.
Add the following to the corresponding files,
//= require components
//= require_self
//= require react_ujs
window.React:require('react');
window.ReactDOM:require('react-dom');
window.SampleComponent:require('./components/sample_component.jsx');
For testing we're be creating a sample component which will be placed inside the components folder and has the following content,
var SampleComponent:React.createClass({
render: function () {
return (
<div>
<h3>This is a sample testing component</h3>
<p>The title prop you passed is: {this.props.title}</p>
</div>
);
},
});
We're almost there! Now you can define all the components you want, just like this. Lets try using it.
rails g controller home index
This will create a new controller and action home#index so you can view it from app/views/home/index.html.erb.
Now you can render the SampleComponent in the view by adding this to app/views/home/index.html.erb
<%= react_component "SampleComponent", { title: "A sample title" } %>
Then start the Rails server and navigate to http://localhost:3000/home/index.
If you can see something similar to the below screen, there then you've installed Browserify! :)
Hope the article helps you fixing browserify and react for your rails application. Please feel free to comment down below if you've got any queries.
Also I've published the source code on github.com/varun, clone and have your way with it!
Last updated: January 23rd, 2024 at 1:50:36 PM GMT+0