devblockchain
Setting up your development environment for Hyperledger Fabric
Get a peek into how a professional Hyperledger Fabric dev environment looks like. We take you from the directory structure to custom scripts we've written to automate testing.

Karthik Kamalakannan / 03 March, 2018
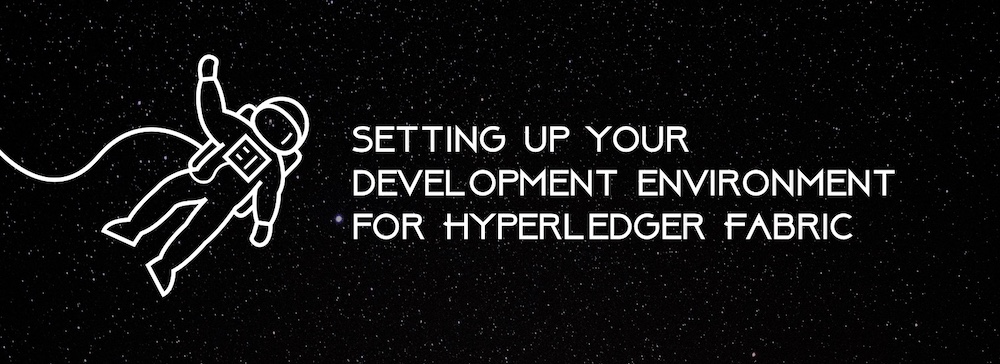
The Directory Structure
Having a clean directory structure enables Fabric programmers to instinctively know where each file should sit. Here is our approach to organising the Fabric dev environment.
Where should the Chaincode go?
A lot of developers, mount the chaincode directly from the development folder - that's a terrible idea.
What you should do is develop chaincode in your GOPATH under the vendor-name github.com/yourproject/and-here-goes-your-chaincode-files
. You can also write a script which will copy your chaincode under GOPATH and run a go build command to spit out the go-errors before running it in your Fabric tools. This will reduce your development to production time.
Here's a simple script to copy your chaincode,
#!/bin/bash
echo "Copying your chaincode to the place where it belongs."
rm -rf $GOPATH/src/github.com/project-name/go & mkdir -p $GOPATH/src/github.com/project-name/go
cp -Rf chaincode/* $GOPATH/src/github.com/project-name/go/
If you want to build the code, just add this,
#!/bin/bash
echo "Stepping into the chaincode's directory"
cd $GOPATH/src/github.com/acmebc/go/
echo "Building your chaincode. Tada."
go build .
This will build once when you finish your chaincode development. It will deal with all the 'unused-variables', 'scope-problems' and any other Go warnings.
Starting Your Network
Let's assume that your Fabric network is up and running. Ok? What if you didn't have a network running? Yeah. I can understand.
I am keeping this article as simple as possible. So, follow these steps as of now. The deep philosophies behind every single step will be explained later.
git clone https://github.com/hyperledger/fabric-samples
If you don't have Fabric installed in your environment,
cd fabric-samples/scripts ./fabric-preload.sh
Then proceed with this,
cd fabric-samples/basic-network vi docker-compose.yml
Under CLI services, see the volumes mounted. You can see that the chaincode is mounted with a relative folder. Let's change it,
cli:
container_name: cli
image: hyperledger/fabric-tools
tty: true
environment:
- GOPATH=/opt/gopath
- CORE_VM_ENDPOINT=unix:///host/var/run/docker.sock
- CORE_LOGGING_LEVEL=DEBUG
- CORE_PEER_ID=cli
- CORE_PEER_ADDRESS=peer0.org1.example.com:7051
- CORE_PEER_LOCALMSPID=Org1MSP
- CORE_PEER_MSPCONFIGPATH=/opt/gopath/src/github.com/hyperledger/fabric/peer/crypto/peerOrganizations/org1.example.com/users/Admin@org1.example.com/msp
- CORE_CHAINCODE_KEEPALIVE=10
working_dir: /opt/gopath/src/github.com/hyperledger/fabric/peer
command: /bin/bash
volumes:
- /var/run/:/host/var/run/
- $GOPATH/src/github.com/:/opt/gopath/src/github.com/
- ./crypto-config:/opt/gopath/src/github.com/hyperledger/fabric/peer/crypto/
networks:
- basic
If you see at Line 18, it has been changed with respect to GOPATH. 😄
Now let's start our docker-compose i.e, our network with our chaincode mounted to it.
Let's do it in our style. Create a bash file named start-network.sh,
#!/bin/bash
start-network.sh
cd basic-network ./start.sh
Now the fabric-network is up and running. You can check it by typing docker ps in console.
Installing the Chaincode
Now our time to launch our armageddon. So we have our network running. Lets install the chaincode using the bash script we wrote,
#!/bin/bash
# install-chaincode.sh
LANGUAGE=golang
CC_SRC_PATH=github.com/acmebc/go
CC_VERSION=1.0
docker exec -e "CORE_PEER_LOCALMSPID=Org1MSP" -e "CORE_PEER_MSPCONFIGPATH=/opt/gopath/src/github.com/hyperledger/fabric/peer/crypto/peerOrganizations/org1.example.com/users/[email protected]/msp" cli peer chaincode install -n acmebc -v $CC_VERSION -p "$CC_SRC_PATH" -l "$LANGUAGE"
docker exec -e "CORE_PEER_LOCALMSPID=Org1MSP" -e "CORE_PEER_MSPCONFIGPATH=/opt/gopath/src/github.com/hyperledger/fabric/peer/crypto/peerOrganizations/org1.example.com/users/[email protected]/msp" cli peer chaincode instantiate -o orderer.example.com:7050 -C mychannel -n acmebc -l "$LANGUAGE" -v $CC_VERSION -c '{"Args":\\\[""\\\]}' -P "OR ('Org1MSP.member')"
That's all! You might be wondering, why do we need to write a bash script for every small process? Answer to that question: You can write a single bash master script to control everything with logical-conditions.
Now that we have our chaincode installed. You can try the query/invoke commands and check how it's working. Or you can ping the application that you integrated with the network.
Updating the Chaincode
This is where developers waste time. Updating the code, building it and deploying it again by updating the chaincode-version. Doing it in our style, let's write a script.
#!/bin/bash
LANGUAGE=golang
CC_SRC_PATH=github.com/acmebc/go
CC_VERSION=1.0
TEMP_CC_VERSION+=$(date +".%I.%M.%S") # Appending with temporary timestamp.
# Replace the chaincode with our new updated code.
rm -rf $GOPATH/src/github.com/project-name/go & mkdir -p $GOPATH/src/github.com/project-name/go
cp -Rf chaincode/\\\* $GOPATH/src/github.com/project-name/go/
# Building the go chaincode
cd $GOPATH/src/github.com/project-name/go/
go build .
# Run the update action
docker exec -e "CORE_PEER_LOCALMSPID=Org1MSP" -e "CORE_PEER_MSPCONFIGPATH=/opt/gopath/src/github.com/hyperledger/fabric/peer/crypto/peerOrganizations/org1.example.com/users/[email protected]/msp" cli peer chaincode install -n acmebc -v $TEMP_CC_VERSION -p "$CC_SRC_PATH" -l "$LANGUAGE"
docker exec -e "CORE_PEER_LOCALMSPID=Org1MSP" -e "CORE_PEER_MSPCONFIGPATH=/opt/gopath/src/github.com/hyperledger/fabric/peer/crypto/peerOrganizations/org1.example.com/users/[email protected]/msp" cli peer chaincode upgrade -o orderer.example.com:7050 -C mychannel -n acmebc -l "$LANGUAGE" -v $TEMP_CC_VERSION -c '{"Args":\\\["init"\\\]}' -P "OR ('Org1MSP.member')"
That's it. Updated, yo! Run your queries and see the magic.
Click here to learn more about Hyperleger and Blockchain straight from our developers.
Last updated: January 23rd, 2024 at 1:50:36 PM GMT+0